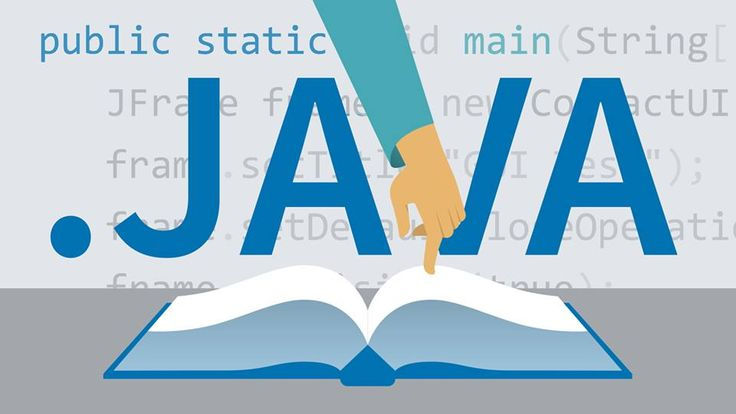
Java is renowned for its versatility and robustness, but writing clean and efficient Java code requires more than just knowing the syntax. It involves adhering to best practices that enhance readability, maintainability, and performance. Here are some essential tips for writing clean and efficient Java code.
1. Follow Naming Conventions
Consistent naming conventions are crucial for readability and understanding. In Java, use camelCase for variables and methods, such as calculateSum or totalAmount, and PascalCase for classes, like CustomerAccount or OrderDetails. Constants should be in uppercase letters with underscores, for example, MAX_HEIGHT or DEFAULT_TIMEOUT.
2. Write Small and Focused Methods
Methods should be small and focused, performing a single task. Large, complex methods are difficult to understand and maintain. If a method exceeds 20-30 lines, consider breaking it down into smaller, more manageable pieces.
3. Use Meaningful Variable and Method Names
Choose names that clearly describe the purpose of the variable or method. Avoid single-letter names, except for temporary variables in loops. For instance, instead of naming a method process, name it processOrder to clarify its function.
4. Favor Composition Over Inheritance
Inheritance can lead to tightly coupled and fragile code, while composition offers flexibility and reusability. Composition involves creating complex objects by combining simpler ones, making your code easier to modify and extend.
5. Use Interfaces and Abstract Classes Appropriately
Interfaces and abstract classes serve different purposes. Interfaces define a contract that classes must adhere to, while abstract classes provide a base with some shared implementation. Use interfaces to define behaviors that classes should implement, and abstract classes when sharing common code among related classes.
6. Encapsulate Fields and Use Getters and Setters
Encapsulation is a key principle of object-oriented programming. Make fields private and provide public getter and setter methods to access and modify them. This practice protects the internal state of your objects and allows you to control how data is accessed or modified.
7. Avoid Magic Numbers
Magic numbers are hard-coded values with no explanation. Replace them with named constants to make your code more readable and maintainable. For example, instead of using an unexplained number directly in your code, define a named constant at the beginning of your class.
8. Use Enum Types Instead of Constant Integers or Strings
Enums provide a type-safe way to represent a fixed set of constants with meaningful names. They can also include fields, methods, and constructors, offering more functionality and clarity than simple integer or string constants.
9. Handle Exceptions Properly
Exceptions should handle error conditions, not normal control flow. Catch specific exceptions rather than the generic Exception class, and clean up resources in a finally block or use the try-with-resources statement for automatic resource management.
10. Use Streams and Lambda Expressions
Introduced in Java 8, streams and lambda expressions provide a functional approach to processing collections, making your code more concise and readable. Streams allow you to perform operations like filtering and mapping on data in a declarative manner.
11. Optimize Performance with Caution
While performance is important, premature optimization can make your code complex and hard to maintain. Focus first on writing clear and correct code. Use profiling tools to identify performance bottlenecks and optimize only the critical parts of your code.
12. Leverage Java's Standard Libraries
Java's standard libraries offer a wide range of well-tested and optimized functionalities. Reuse these libraries instead of writing your own implementations for common tasks, as this can save time and reduce the likelihood of bugs.
13. Use Annotations and Avoid Reflection
Annotations provide a way to add metadata to your code, making it more expressive and less error-prone. Reflection, on the other hand, can lead to performance issues and brittle code, so it should be avoided unless absolutely necessary.
14. Write Unit Tests
Unit tests ensure that your code works as expected and help prevent future bugs. Use a testing framework like JUnit to write and run your tests. Aim for high test coverage, balancing it with the need for maintainable and understandable tests.
15. Document Your Code
While clean code should be self-explanatory, comments are still valuable for explaining why certain decisions were made. Use documentation comments to describe the purpose and usage of classes and methods. Avoid redundant comments that simply restate what the code does.
16. Practice Code Reviews
Code reviews are an effective way to maintain code quality. They provide an opportunity for team members to share knowledge, catch bugs, and ensure that code adheres to best practices. Encourage a culture of constructive feedback and continuous improvement.
17. Keep Learning
"The Java ecosystem is constantly evolving, with new features and best practices emerging regularly. Stay up-to-date by reading books, articles, and documentation, and by participating in the Java community through forums, conferences, and user groups.
By following these tips, you can write Java code that is not only clean and efficient but also maintainable and scalable. Remember, writing good code is an ongoing process that requires continuous learning and adaptation. Consider enrolling in a Java course in Patna, Nagpur, Indore, Delhi, Noida, and other cities in India to deepen your understanding and stay ahead in the ever-changing Java landscape. Happy coding!
Comments